In a previous post, I gave a quick review of Flowgorithm, which is a program that allows users to program with flowcharts.
In this post, I will show how I used Flowgorithm as a scaffolding tool to teach my students how to create an interactive HTML form with Javascript.
Since Javascript is mainly a frontend language for websites, one would need to also know HTML and CSS to use it effectively. Students will need to know the basics of the Document Object Model (DOM) of a webpage and a bit of basic CSS if they want to properly add some style into their webpage.
Students will also need to be able to create programs in flowgorithm.
For this demonstration, I will be showing a simple “hello name” program to illustrate the basic idea of getting input from the form and displaying the output in a div element on the page.
Create HTML Page
This program is fairly simple. The user inputs their name and the program will output “Hello <name>!” where <name> is replace with whatever the user input.
Before we look at the flowchart, let’s take a look at what the HTML page and form would look like.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Hello name!</title>
</head>
<body>
<form>
<label>Enter your name: </label>
<input type="text" id="name">
<input type="submit">
</form>
<div id="output"></div>
</body>
</html>
Creating Flowchart
The student will need to know how to create a variable, get input, and display output of a variable. The flowchart for flowgorithm is displayed below.
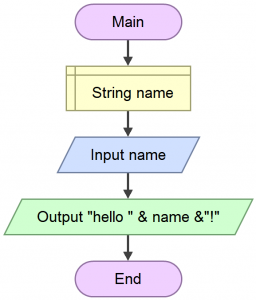
Generating Javascript
The Javascript flowgorithm produces from the flowchart is shown below.
function Main() {
var name;
name = input("Name");
output("hello " + name + "!");
}
/**
The following functions are called whenever
the program needs to input or output data.
You can change these functions to use other
webpage elements rather than pop-up windows.
*/
function input (name) {
return window.prompt("Please enter a value for " + name, "");
}
function output (text) {
window.alert(text);
}
Combine Javascript and HTML
Notice that the Javascript program is contained in a function called main. Since it’s contained in a function, we can either put it into the head or the body of the HTML document. In this tutorial, we will place it in the head of the HTML document. The HTML document should now look as below with modifications highlighted:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Hello name!</title>
<script>
function Main() {
var name;
name = input("Name");
output("hello " + name + "!");
}
/**
The following functions are called whenever
the program needs to input or output data.
You can change these functions to use other
webpage elements rather than pop-up windows.
*/
function input (name) {
return window.prompt("Please enter a value for " + name, "");
}
function output (text) {
window.alert(text);
}
</script>
</head>
<body>
<form>
<label>Enter your name: </label>
<input type="text" id="name">
<input type="submit">
</form>
<div id="output"></div>
</body>
</html>
Link Javascript With Form
The first thing we are going to do is to connect the Javacript code to the HTML form. To do that, we do two things
- set the form action to javascript
- set the submit button to call the main method using the onClick property
The changes are in the lines of code that are highlighted below
<!DOCTYPE html>
<html lang="en">
<head>
<title>Hello name!</title>
<script>
function Main() {
var name;
name = input("Name");
output("hello " + name + "!");
}
/**
The following functions are called whenever
the program needs to input or output data.
You can change these functions to use other
webpage elements rather than pop-up windows.
*/
function input (name) {
return window.prompt("Please enter a value for " + name, "");
}
function output (text) {
window.alert(text);
}
</script>
</head>
<body>
<form action="javascript:">
<label>Enter your name: </label>
<input type="text" id="name">
<input type="submit" onClick="Main();">
</form>
<div id="output"></div>
</body>
</html>
When you click the submit button, a prompt should display. You can type the your name. Then a Javascript alert box will appear saying “Hello <name>!”
Get input from form instead of input window
We do not want to use a popup prompt to get the name. Instead, we will use the form we have created. To do so, we just need to change the Javascript code to get the value from the textbox. We use the Document Object Model to get the information with the line below.
document.getElementById(<id goes here>).value;
The script is modified below on the highlighted lines.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Hello name!</title>
<script>
function Main() {
var name;
name = input("name"); // made name to lowercase
output("hello " + name + "!");
}
/**
The following functions are called whenever
the program needs to input or output data.
You can change these functions to use other
webpage elements rather than pop-up windows.
*/
function input (name) {
return document.getElementById(name).value;
}
function output (text) {
window.alert(text);
}
</script>
</head>
<body>
<form action="javascript:">
<label>Enter your name: </label>
<input type="text" id="name">
<input type="submit" onClick="Main();">
</form>
<div id="output"></div>
</body>
</html>
If you refresh the webpage and type your name into the textbox, when you submit, you should only see “Hello <name>!” in the alert box.
Display output on webpage instead of alert box
Just like how we don’t want to input into a prompt, we do not want the result to be displayed in an alert box either.
Notice in our HTML page, we have a DIV element named output. We will put the results there by changing it’s inner HTML with the following lines of Javascript:
document.getElementById("output").innerHTML="Hello " + name + "!";
The above line is inserted into our page on the highlighted line
<!DOCTYPE html>
<html lang="en">
<head>
<title>Hello name!</title>
<script>
function Main() {
var name;
name = input("name"); // made name to lowercase
output("hello " + name + "!");
}
/**
The following functions are called whenever
the program needs to input or output data.
You can change these functions to use other
webpage elements rather than pop-up windows.
*/
function input (name) {
return document.getElementById(name).value;
}
function output (text) {
document.getElementById("output").innerHTML = text;
}
</script>
</head>
<body>
<form action="javascript:">
<label>Enter your name: </label>
<input type="text" id="name">
<input type="submit" onClick="Main();">
</form>
<div id="output"></div>
</body>
</html>
If you refresh the webpage, fill the textbox and click submit, you should see “Hello <name>!” below the form.
Conclusion
I hope this tutorial has given you an idea of how you can use Flowgorithm as a scaffold tool to create an interactive website using Javascript. For my students, they learned up to loops with Flowgorithm before I introduced Javascript to them.
I will make another post in the future with a more advanced use case of scaffolding with Flowgorithm.